Step-by-Step Tutorial: Deploy Node.js and MongoDB to AWS ECS with SonarQube CI/CD Integration
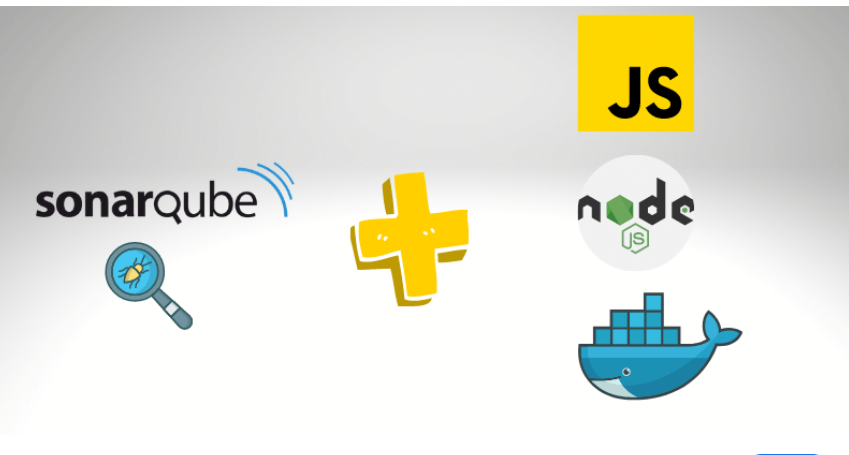
Integrating a Node.js application with MongoDB into AWS Elastic Container Service (ECS) and incorporating SonarQube for code quality checks within a CI/CD pipeline involves several steps. Here’s an outline of the process, including sample code snippets to get you started.
Step 1: Containerize the Node.js Application
- Create a Dockerfile in the root of your Node.js project. This file defines the environment for running your application.
# Use an official Node runtime as a parent image
FROM node:14
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy the current directory contents into the container at WORKDIR
COPY . .
# Install any needed packages specified in package.json
RUN npm install
# Make port 3000 available to the world outside this container
EXPOSE 3000
# Define environment variable
ENV NAME World
# Run app.js when the container launches
CMD ["node", "app.js"]
Step 2:Build your Docker image using the following command:
docker build -t my-nodejs-app .
Step 2: Push the Docker Image to a Repository
- Tag your image to match your repository name.
docker tag my-nodejs-app:latest myrepository/my-nodejs-app:latest
2.Push your Docker image to a container registry like Docker Hub or AWS ECR (Elastic Container Registry).
docker push myrepository/my-nodejs-app:latest
Step 3: Create a MongoDB Instance
You can use a managed MongoDB service like Amazon DocumentDB or MongoDB Atlas, or you can containerize MongoDB yourself.
You can use a managed MongoDB service like Amazon DocumentDB or MongoDB Atlas, or you can containerize MongoDB yourself.
Step 4: Set Up AWS ECS
- Create an ECS cluster.
- Define a task definition that includes your Node.js application and (optionally) your MongoDB container if you’re not using a managed service.
- Configure a service within the ECS cluster to run and manage the tasks.
Step 5: Set Up CI/CD Pipeline with AWS CodePipeline
- Source Stage: Use CodeCommit, GitHub, or Bitbucket as your source repository.
- Build Stage: Use AWS CodeBuild to build your Docker image and push it to ECR. Here you can also integrate SonarQube for code quality checks.BuildSpec.yml example for AWS CodeBuild:
version: 0.2
phases:
pre_build:
commands:
- echo Logging in to Amazon ECR...
- $(aws ecr get-login --no-include-email --region $AWS_DEFAULT_REGION)
- REPOSITORY_URI=your_ecr_repository_url
- COMMIT_HASH=$(echo $CODEBUILD_RESOLVED_SOURCE_VERSION | cut -c 1-7)
- IMAGE_TAG=${COMMIT_HASH:=latest}
build:
commands:
- echo Build started on `date`
- echo Building the Docker image...
- docker build -t $REPOSITORY_URI:$IMAGE_TAG .
- docker tag $REPOSITORY_URI:$IMAGE_TAG $REPOSITORY_URI:$IMAGE_TAG
post_build:
commands:
- echo Build completed on `date`
- echo Pushing the Docker image...
- docker push $REPOSITORY_URI:$IMAGE_TAG
- echo Writing image definitions file...
- printf '[{"name":"container-name","imageUri":"%s"}]' $REPOSITORY_URI:$IMAGE_TAG > imagedefinitions.json
artifacts:
files: imagedefinitions.json
- Deploy Stage: Use AWS ECS as the deployment provider. The
imagedefinitions.json
file created during the build stage will be used to update the ECS service with the new Docker image.
Step 6: Integrate SonarQube for Code Quality Checks
You can add a step in your buildspec.yml
to run SonarQube analysis. This typically involves installing and configuring the SonarScanner CLI in your build environment and then running the scanner against your codebase.
phases:
pre_build:
commands:
- echo Running SonarQube analysis...
- sonar-scanner \
-Dsonar.projectKey=my-nodejs-app \
-Dsonar.sources=. \
-Dsonar.host.url=http://mySonarQubeServer.com \
-Dsonar.login=mySonarQubeToken
Ensure that SonarQube is configured to analyze your project, and you have created a project key in SonarQube that matches what you specify in the buildspec.yml
.
Please refer the github code and already hosted on the localmachine :
Nodejs on Local Machine with Mongodb database
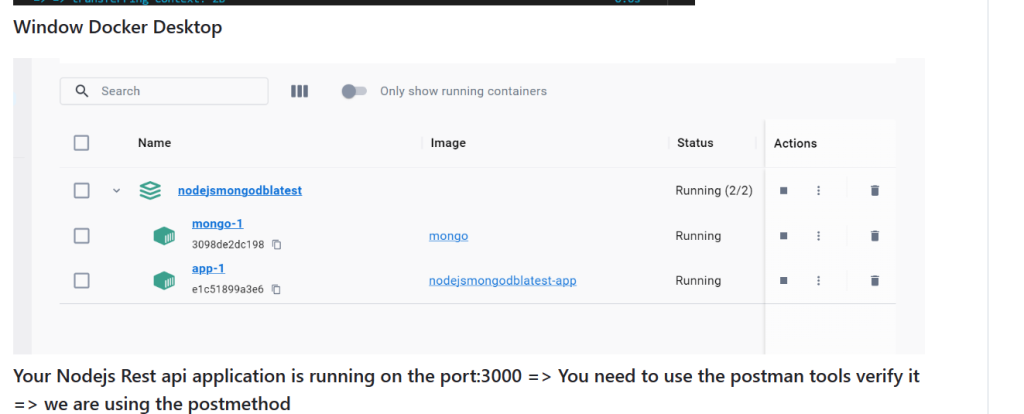