AWS Lambda and Business Use Case
AWS Lambda is a serverless computing service provided by Amazon Web Services (AWS) that allows you to run code without provisioning or managing servers. As a beginner in AWS solution architecture, understanding the basics of Lambda can help you design and implement scalable and cost-effective solutions. Here’s an overview of AWS Lambda for beginners:
AWS Lambda Integaration with AWS Api Gateway

- Serverless Computing:
- Traditional computing requires managing servers, scaling capacity, and optimizing resource utilization.
- Serverless computing abstracts away the infrastructure management, allowing you to focus on writing code and building applications.
- With Lambda, you only pay for the actual compute time your code consumes, eliminating idle server costs.
- Key Concepts:
- Lambda Function: It’s the code you write and deploy to AWS Lambda. Functions are triggered by events and automatically scale to handle incoming requests.
- Event Sources: Lambda functions can be triggered by various events, such as API Gateway requests, file uploads to S3, changes in DynamoDB tables, or messages in an SQS queue.
- Execution Environment: AWS Lambda provides a runtime environment for your code, supporting multiple programming languages like Node.js, Python, Java, and more.
- Concurrency and Scaling: Lambda automatically scales your functions to handle the incoming workload, and you can configure the concurrency limits.
- Benefits of AWS Lambda:
- Scalability: Lambda scales your functions in response to incoming requests, ensuring high availability and performance without manual intervention.
- Cost-effectiveness: You are billed based on the actual execution time of your code, without incurring costs for idle servers.
- Operational Simplicity: AWS manages the infrastructure, including patching, scaling, and availability, allowing you to focus on writing code.
- Event-driven Architecture: Lambda integrates seamlessly with various AWS services, enabling event-driven application architectures.
- Use Cases for AWS Lambda:
- API Backends: Build scalable and serverless APIs using Lambda and API Gateway, allowing you to handle millions of requests without managing infrastructure.
- Data Processing: Process and transform data in real-time or batch jobs, such as image or video processing, log analysis, or data validation.
- Microservices: Implement microservice architectures by breaking down your application into small, independent Lambda functions.
- IoT: Handle events from IoT devices, process sensor data, and trigger actions based on device events.
- Chatbots and Voice Assistants: Develop conversational interfaces by integrating Lambda with messaging platforms or voice services like Amazon Lex.
- Getting Started:
- Create an AWS account and access the AWS Management Console.
- Use the AWS Lambda service to create and configure your Lambda functions.
- Write your function code or upload an existing code package.
- Set up event sources to trigger your Lambda functions.
- Test your functions using the Lambda console or invoke them programmatically.
- Monitor and troubleshoot your functions using CloudWatch Logs and metrics.
- Best Practices:
- Function Design: Design functions to be stateless, idempotent, and modular. Leverage environment variables and function configuration effectively.
- Error Handling: Implement appropriate error handling and retries in your functions.
- Security: Apply AWS IAM roles and policies to grant necessary permissions to your functions.
- Logging and Monitoring: Use CloudWatch Logs and metrics to gain visibility into the execution and performance of your functions.
- Deployment Automation: Use AWS SAM (Serverless Application Model) or other deployment frameworks to automate the deployment of your functions.
AWS Lambda provides a powerful and scalable platform for building serverless applications. By understanding the core concepts, benefits, use cases, and best practices of Lambda, you can leverage this service to design and implement scalable, cost-efficient, and event-driven architectures in your AWS solutions.
AWS Lambda Sample code with AWS Api Gateway via Dyanamodb
how you can create an AWS Lambda function with .NET Core, integrate it with AWS API Gateway, and interact with DynamoDB:
Prerequisites:
- AWS account with API Gateway, Lambda, and DynamoDB services set up.
- AWS CLI or AWS Management Console access for configuration.
Step 1: Set Up DynamoDB:
- Open the AWS Management Console and navigate to the DynamoDB service.
- Create a table with the desired configuration, such as table name and primary key.
- Make note of the table name and primary key for later use.
Step 2: Create a .NET Core Lambda Project:
- Install .NET Core SDK on your machine if not already installed.
- Open a terminal or command prompt and run the following command to create a new Lambda project:arduinoCopy code
dotnet new lambda.Empty -n MyLambdaProject cd MyLambdaProject
- dotnet new lambda.Empty -n MyLambdaProject
- cd MyLambdaProject
Step 3: Add Dependencies and Code to the Lambda Function:
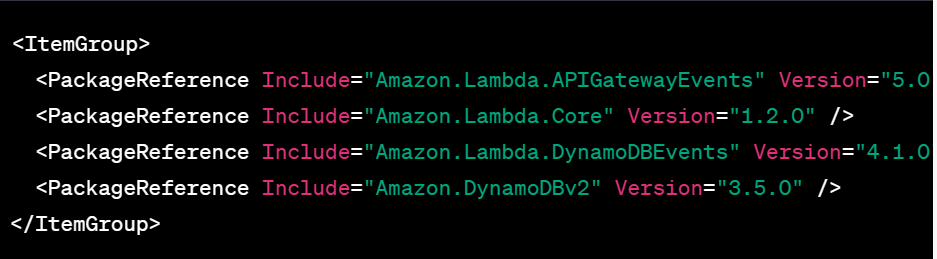
Replace the contents of Function.cs with the following code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Amazon.Lambda.APIGatewayEvents;
using Amazon.Lambda.Core;
using Amazon.DynamoDBv2;
using Amazon.DynamoDBv2.DocumentModel;
[assembly: LambdaSerializer(typeof(Amazon.Lambda.Serialization.SystemTextJson.DefaultLambdaJsonSerializer))]
namespace MyLambdaProject
{
public class Function
{
private readonly IAmazonDynamoDB _dynamoDbClient;
private const string TableName = “YourDynamoDbTableName”;
public Function()
{
_dynamoDbClient = new AmazonDynamoDBClient();
}
public async Task<APIGatewayProxyResponse> FunctionHandler(APIGatewayProxyRequest request, ILambdaContext context)
{
try
{
var document = new Document();
document["id"] = Guid.NewGuid().ToString();
document["message"] = "Hello from AWS Lambda!";
var table = Table.LoadTable(_dynamoDbClient, TableName);
await table.PutItemAsync(document);
return new APIGatewayProxyResponse
{
StatusCode = 200,
Body = "Item added to DynamoDB",
Headers = new Dictionary<string, string> { { "Content-Type", "text/plain" } }
};
}
catch (Exception ex)
{
return new APIGatewayProxyResponse
{
StatusCode = 500,
Body = $"Error: {ex.Message}",
Headers = new Dictionary<string, string> { { "Content-Type", "text/plain" } }
};
}
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Amazon.Lambda.APIGatewayEvents;
using Amazon.Lambda.Core;
using Amazon.DynamoDBv2;
using Amazon.DynamoDBv2.DocumentModel;
[assembly: LambdaSerializer(typeof(Amazon.Lambda.Serialization.SystemTextJson.DefaultLambdaJsonSerializer))]
namespace MyLambdaProject
{
public class Function
{
private readonly IAmazonDynamoDB _dynamoDbClient;
private const string TableName = “YourDynamoDbTableName”;
public Function()
{
_dynamoDbClient = new AmazonDynamoDBClient();
}
public async Task<APIGatewayProxyResponse> FunctionHandler(APIGatewayProxyRequest request, ILambdaContext context)
{
try
{
var document = new Document();
document["id"] = Guid.NewGuid().ToString();
document["message"] = "Hello from AWS Lambda!";
var table = Table.LoadTable(_dynamoDbClient, TableName);
await table.PutItemAsync(document);
return new APIGatewayProxyResponse
{
StatusCode = 200,
Body = "Item added to DynamoDB",
Headers = new Dictionary<string, string> { { "Content-Type", "text/plain" } }
};
}
catch (Exception ex)
{
return new APIGatewayProxyResponse
{
StatusCode = 500,
Body = $"Error: {ex.Message}",
Headers = new Dictionary<string, string> { { "Content-Type", "text/plain" } }
};
}
}
}
}
Step 4: Publish the Lambda Function:
- Run the following command to publish the Lambda function:javascriptCopy code
dotnet lambda deploy-function
Step 5: Create an API Gateway and Configure Integration:
- Open the AWS Management Console and navigate to the API Gateway service.
- Create a new API and configure the desired settings.
- Create a new resource and method (e.g., POST) for your API.
- Configure the integration type as “Lambda Function” and select the Lambda function you deployed in Step 4.
- Save the changes.
Step 6: Test the Integration:
- In the API Gateway console, navigate to the resource and method you created.
- Click on “Test” and provide a sample request.
- Verify that the API Gateway successfully invokes the Lambda function, and the response indicates that the item was added to DynamoDB.
Congratulations! You have successfully created an AWS Lambda function with .NET Core, integrated it with API Gateway, and interacted with DynamoDB. You can now further customize and enhance the Lambda function and API Gateway configuration to meet your specific requirements.
Here’s a sample code snippet that demonstrates how to create an AWS Lambda function using .NET Core and utilize DynamoDB as an event source:
using System.Collections.Generic;
using System.Threading.Tasks;
using Amazon.DynamoDBv2;
using Amazon.DynamoDBv2.Model;
using Amazon.Lambda.Core;
using Amazon.Lambda.DynamoDBEvents;
[assembly: LambdaSerializer(typeof(Amazon.Lambda.Serialization.SystemTextJson.DefaultLambdaJsonSerializer))]
namespace DynamoDBEventExample
{
public class Function
{
private readonly IAmazonDynamoDB _dynamoDbClient;
public Function()
{
_dynamoDbClient = new AmazonDynamoDBClient();
}
public async Task FunctionHandler(DynamoDBEvent dynamoEvent, ILambdaContext context)
{
foreach (var record in dynamoEvent.Records)
{
if (record.EventName == OperationType.INSERT)
{
// Process inserted item
var newItem = DeserializeItem(record.Dynamodb.NewImage);
// Perform your custom logic with the new item
await ProcessNewItem(newItem);
}
else if (record.EventName == OperationType.MODIFY)
{
// Process modified item
var oldItem = DeserializeItem(record.Dynamodb.OldImage);
var newItem = DeserializeItem(record.Dynamodb.NewImage);
// Perform your custom logic with the old and new items
await ProcessModifiedItem(oldItem, newItem);
}
else if (record.EventName == OperationType.REMOVE)
{
// Process removed item
var oldItem = DeserializeItem(record.Dynamodb.OldImage);
// Perform your custom logic with the old item
await ProcessRemovedItem(oldItem);
}
}
}
private YourItemType DeserializeItem(Dictionary<string, AttributeValue> item)
{
// Deserialize the DynamoDB item into your custom object type
// Customize this method based on your specific item structure
var newItem = new YourItemType();
newItem.Id = item["Id"].S;
newItem.Name = item["Name"].S;
return newItem;
}
private async Task ProcessNewItem(YourItemType newItem)
{
// Perform your custom logic with the new item
// Example: Write the item to a log or send a notification
await Task.CompletedTask;
}
private async Task ProcessModifiedItem(YourItemType oldItem, YourItemType newItem)
{
// Perform your custom logic with the old and new items
// Example: Compare the changes and trigger an action accordingly
await Task.CompletedTask;
}
private async Task ProcessRemovedItem(YourItemType oldItem)
{
// Perform your custom logic with the old item
// Example: Archive the item or update related records
await Task.CompletedTask;
}
}
public class YourItemType
{
public string Id { get; set; }
public string Name { get; set; }
// Add other properties as needed
}
}
In this sample code, the FunctionHandler
method is the entry point for the AWS Lambda function. It takes a DynamoDBEvent
as input, which represents the event generated by DynamoDB. The method iterates over the event records and performs custom logic based on the event type (insert, modify, or remove).
You can customize the YourItemType
class to match your DynamoDB item structure. The DeserializeItem
method demonstrates how to convert the DynamoDB item into your custom object type.
Inside the ProcessNewItem
, ProcessModifiedItem
, and ProcessRemovedItem
methods, you can implement your specific business logic to process the new, modified, or removed items. This could include actions such as logging, sending notifications, updating related records, or triggering other events based on the changes in DynamoDB.
Remember to configure the appropriate AWS Lambda execution role with the necessary permissions to access DynamoDB and process the events.
Note that this is a simplified example, and you may need to adapt it to your specific use case and item structure in DynamoDB.
Certainly! Here’s a sample code snippet that demonstrates an event-driven architecture using AWS Lambda with dotnetcore
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
using Amazon.Lambda.Core;
using Amazon.Lambda.SNSEvents;
[assembly: LambdaSerializer(typeof(Amazon.Lambda.Serialization.SystemTextJson.DefaultLambdaJsonSerializer))]
namespace EventDrivenArchitectureExample
{
public class Function
{
public async Task FunctionHandler(SNSEvent snsEvent, ILambdaContext context)
{
foreach (var record in snsEvent.Records)
{
var message = record.Sns.Message;
var messageId = record.Sns.MessageId;
var timestamp = record.Sns.Timestamp;
// Process the event message
await ProcessEventMessage(message, messageId, timestamp);
}
}
private async Task ProcessEventMessage(string message, string messageId, string timestamp)
{
// Perform your custom logic based on the event message
// Example: Write the message to a log, trigger an action, or update a database
Console.WriteLine($"Received message: {message}");
Console.WriteLine($"MessageId: {messageId}");
Console.WriteLine($"Timestamp: {timestamp}");
await Task.CompletedTask;
}
}
}