How to create the docker file for Nodejs
Creating a Dockerfile for a Node.js application typically involves specifying the environment in which your app will run, copying your app code into the Docker image, and defining how the app should be executed. Here’s a basic example of a Dockerfile for a Node.js app, followed by a line-by-line explanation:
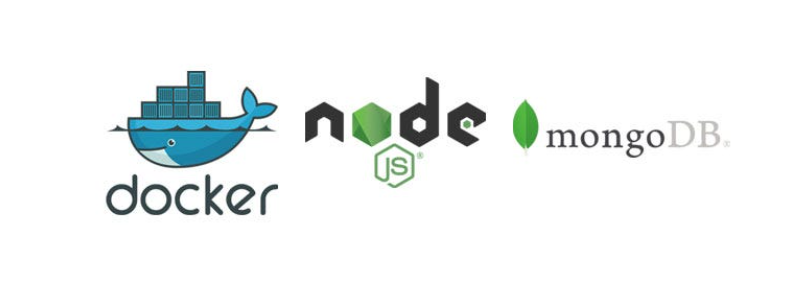
Dockerfile
# 1. Specify the base image
FROM node:14
# 2. Set the working directory in the container
WORKDIR /app
# 3. Copy package.json and package-lock.json
COPY package*.json ./
# 4. Install app dependencies
RUN npm install
# 5. Bundle app source
COPY . .
# 6. Expose the port the app runs on
EXPOSE 3000
# 7. Define the command to run the app
CMD ["npm", "start"]
Now, let’s go through it line by line:
FROM node:14
: This line specifies the base image from which you are building.node:14
means you are using version 14 of Node.js. Docker images for Node.js are pre-built environments that contain Node.js and npm installed. You can choose other versions of Node.js by specifying a different number (e.g.,node:16
).WORKDIR /app
: Sets the working directory inside your Docker container. All subsequent commands will be run from this directory. If it does not exist, Docker will create it for you.COPY package*.json ./
: Copies bothpackage.json
andpackage-lock.json
(if available) to the working directory in the Docker image. This is done before copying the rest of the application to take advantage of Docker’s cache layers – if your dependencies don’t change, Docker can skip the npm install step in subsequent builds, making them faster.RUN npm install
: Executes the command to install your application’s dependencies as defined inpackage.json
. This is a crucial step that prepares your app to be run in the container.COPY . .
: Copies the rest of your application’s source code into the working directory in the Docker image.EXPOSE 3000
: Informs Docker that the container listens on port 3000 at runtime. Note that this does not actually publish the port. It functions as a documentation between the person who builds the image and the person who runs the container, about which ports are intended to be published.CMD ["npm", "start"]
: Specifies the default command to run when the container starts. In this case, it runsnpm start
, which should be defined in yourpackage.json
file under thescripts
section. This typically starts your Node.js application.
Remember, this Dockerfile is just a starting point. Depending on the specifics of your app, you might need additional steps, such as compiling TypeScript files if you’re using TypeScript, or exposing additional ports if your app uses them