Cheatsheet for Terraform as Infrastructure as code
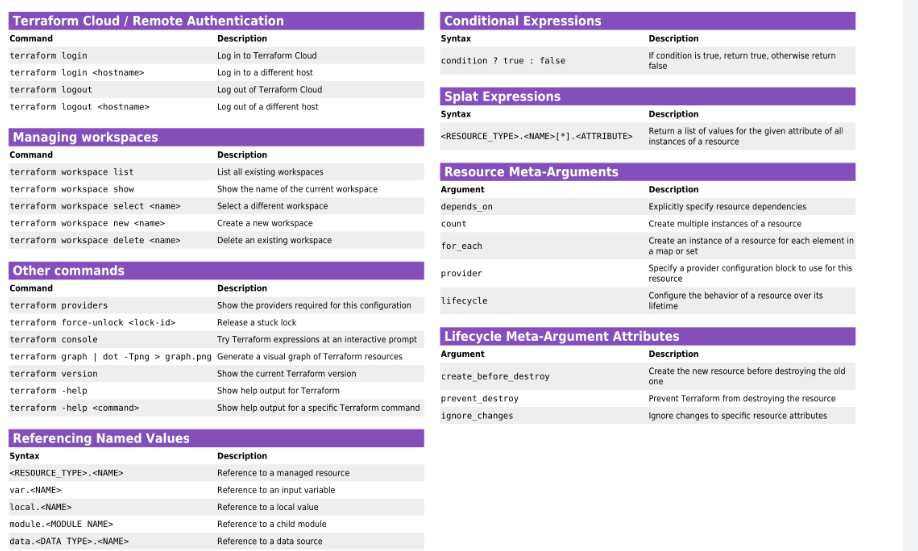
- Definition: Terraform is an open-source tool created by HashiCorp. It allows you to define and provision infrastructure using a high-level configuration language.
- Purpose: It is used to manage cloud services and resources with code, allowing for the automation of deployment and maintenance tasks.
Key Concepts
- Infrastructure as Code (IaC): Manage your physical infrastructure through code similar to how software is managed.
- Providers: Plugins for accessing and managing resources in a specific cloud provider (e.g., AWS, Azure, Google Cloud).
- Resources: Components of your infrastructure, like virtual machines, networks, or databases.
- Modules: Reusable, encapsulated Terraform configurations for organizing and packaging code.
- State: Terraform tracks the state of your managed infrastructure and configuration.
Basic File Structure
main.tf
: Main file for Terraform configurations.variables.tf
: Optional file to define variables.outputs.tf
: Optional file to define outputs.provider.tf
: Optional file to define providers.
Core Terraform Commands
terraform init
: Initialize a Terraform working directory by installing necessary providers.terraform plan
: Create an execution plan, showing what Terraform will do when you apply changes.terraform apply
: Apply changes required to reach the desired state of the configuration.terraform destroy
: Destroy the Terraform-managed infrastructure.
Commonly Used Commands
terraform fmt
: Automatically formats Terraform code to a canonical format.terraform validate
: Validates the configuration for syntax and consistency.terraform output
: Read outputs from your state file.
Best Practices for Beginners
- Version Control: Always store your Terraform configurations in version control systems like Git.
- Module Use: Use modules to organize and reuse code, which can simplify management of larger infrastructures.
- Automate: Use automation tools like Terraform Cloud or CI/CD pipelines to apply Terraform changes safely.
- Secure State Files: Protect and back up your Terraform state files, which contain sensitive data.
- Review Plans: Always review the plan before applying to avoid unintended changes to your infrastructure.
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "ExampleInstance"
}
}
Tips for Debugging
- Use
terraform plan
to see changes without applying them. - Check Terraform’s detailed logs by setting the
TF_LOG
environment variable (e.g.,export TF_LOG=DEBUG
).